Use Case
I need to create an application that requires me to enter command line arguments to a WPF application and print those values or use them within my application.
Implementation
When I create a WPF application I am stuck with the very simple constructor
public MainWindow()
{
InitializeComponent();
}
Rather than the arguments easily accessible to me with the start Main method.
class Program
{
static void Main(string[] args)
{
}
}
In order to access command line arguments in a WPF application I need to access the App Environment, extremely simple to achieve by using one line of code
string[] args = Environment.GetCommandLineArgs();
I can access any value I pass through my application now and args will hold the value. But what if I am after something a little more advanced. Something like
myapplication.exe --message "Hello World"
Ok so what I know here is that the first argument is the --message and the second argument is Hello World - so the next logical thing to do is create a loop that will hold all this information in a key value pair. The best collection I can use for this job is a Dictionary, it allows me to hold a key value pair of two different object types. Our MainWindow
class turns in to something like this:
public partial class MainWindow : Window
{
Dictionary<string, string> arguments = new Dictionary<string, string>();
public MainWindow()
{
InitializeComponent();
string[] args = Environment.GetCommandLineArgs();
for (int index = 1; index < args.Length; index += 2)
{
string arg = args[index].Replace("--", "");
arguments.Add(arg, args[index + 1]);
}
}
}
The loop looks for a string with "--" and replaces it so I can just simply use the argument name in my code. The Dictionary will be populated with the key value pair of my command line arguments.
Running the application
I have gone ahead and created a TextBlock in my MainWindow for the WPF application, I now just need to add some code to change it's value with whatever I enter in my command line arguments.
if (arguments.ContainsKey("message"))
{
txtMessage.Text = arguments["message"];
}
Now when I run my application using the following command line arguments I should see the message displayed in my Window.
myapplication.exe --message "Hello World"
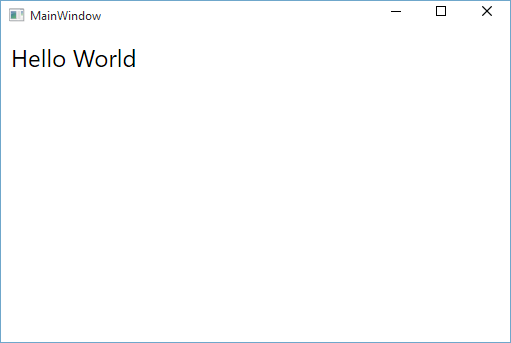
As you can see the Hello World message that was expected to be printed in the window is there as it should be.
Download
You can download the demo application used in this article from my Github repository. This application is provided as is with no support.
Credits
Credit goes to ChrisF over at Stackoverflow for providing the solution.